For this exercise we need to implement the Connected Components Algorithm, to detect the objects in an image.
The input image that we used in this exercise contains 5 different objects:
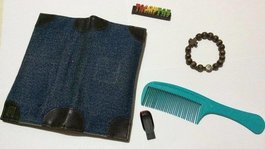
First, we converted the image to a gray scale image and then applied the blur() and medianBlur() to produce a smoother image. Then a threshold() function was applied to convert the grayscale image to a binary image.
After this, we applied the connected components algorithm.
For the connected components, a 2×2 integer array was created and contains 0 as initial values. The algorithm has two passes, for the first pass the image is traversed to check if the pixels is a foreground or a background, in this pass only the black pixels or pixels with a 0 value will be considered. So for each black pixel we check for its top and left neighbors, if the label in the arrlbl of the neighbors is 0.
- If both the top and left pixels are labelled 0, a new label will be created and will be added to the equivalence table.
- If only top pixel is labelled 0, we will copy the label from the left pixel.
- If only left pixel is labelled 0, we will copy the label from the top pixel.
- If both pixels are labelled 1, we will still copy the label from the top pixel; however, we will add the pixel with the larger label to the equivalent row of the smaller label.
For the second pass, the image will be traversed again, background pixels will not be considered. From the last label to the first label. From the equivalence table, higher values are converted to smaller ones based from their equivalence.
After applying the second pass, we now proceed to coloring the image according to their labels. And this is the output of the labelled image:
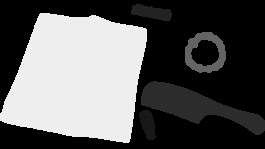
In order to use the rectangle() function to box the labels, we needed the upper-left and the lower-right vertex of each object and use it as parameters for the function. And this is the output image: